|
|
|
This article will show you how to use IP Helper APIs GetAdaptersInfo, GetInterfaceInfo, IPReleaseAddress and IPRenewAddress to list all available IPs and perform renew/release operation on a selected IP.
Before we see actual code lets quickly check the description of each API.
GetAdaptersInfo : This is a very useful function which returns information about all installed adapters on local machine. This function fills IP_ADAPTER_INFO structure with all useful information from which you can create your own IpConfig utility. for more information see my article How to retrive IP Configuration of local machine
GetInterfaceInfo : This function is just like GetAdaptersInfo but it only returns Adapter Name and Index.You can use Adapter index while calling IP Helper functions like IPReleaseAddress and IPRenewAddress.
IPReleaseAddress : The IpReleaseAddress function releases an IP address previously obtained through Dynamic Host Configuration Protocol (DHCP). You have to pass Adapter information using IP_ADAPTER_INDEX_MAP structure which contains Adapter name and index.
IpRenewAddress : The IpRenewAddress function renews a lease on an IP address previously obtained through Dynamic Host Configuration Protocol (DHCP). You have to pass Adapter information using IP_ADAPTER_INDEX_MAP structure which contains Adapter name and index.
Now real fun,
Step-By-Step Example
- Create a standard exe project - Add two command button and one listbox control on the form1 - Add the following code in form1 |
Click here to copy the following block | Private Const MAX_HOSTNAME_LEN = 132 Private Const MAX_DOMAIN_NAME_LEN = 132 Private Const MAX_SCOPE_ID_LEN = 260 Private Const MAX_ADAPTER_NAME_LENGTH = 260 Private Const MAX_ADAPTER_ADDRESS_LENGTH = 8 Private Const MAX_ADAPTER_DESCRIPTION_LENGTH = 132
Private Const ERROR_SUCCESS = 0& Private Const ERROR_BUFFER_OVERFLOW As Long = 111 Private Const ERROR_INSUFFICIENT_BUFFER As Long = 122
Private Const GMEM_FIXED As Long = &H0
Private Type IP_ADDR_STRING Next As Long IpAddress As String * 16 IpMask As String * 16 Context As Long End Type
Private Type IP_ADAPTER_INFO Next As Long ComboIndex As Long AdapterName As String * MAX_ADAPTER_NAME_LENGTH Description As String * MAX_ADAPTER_DESCRIPTION_LENGTH AddressLength As Long Address(MAX_ADAPTER_ADDRESS_LENGTH - 1) As Byte Index As Long Type As Long DhcpEnabled As Long CurrentIpAddress As Long IpAddressList As IP_ADDR_STRING GatewayList As IP_ADDR_STRING DhcpServer As IP_ADDR_STRING HaveWins As Byte PrimaryWinsServer As IP_ADDR_STRING SecondaryWinsServer As IP_ADDR_STRING LeaseObtained As Long LeaseExpires As Long End Type
Private Type IP_ADAPTER_INDEX_MAP Index As Long AdapterName(0 To MAX_ADAPTER_NAME_LENGTH - 1) As Integer End Type
Private Type IP_INTERFACE_INFO NumAdapters As Long Adapter As IP_ADAPTER_INDEX_MAP End Type
Private Declare Function GetAdaptersInfo Lib "IPHLPAPI.dll" ( _ IpAdapterInfo As Any, _ pOutBufLen As Long) As Long
Private Declare Function GetInterfaceInfo Lib "IPHLPAPI.dll" ( _ ByVal pIfTable As Long, _ dwOutBufLen As Long) As Long
Private Declare Function IPReleaseAddress Lib "IPHLPAPI.dll" Alias "IpReleaseAddress" ( _ AdapterInfo As IP_ADAPTER_INDEX_MAP) As Long
Private Declare Function IPRenewAddress Lib "IPHLPAPI.dll" Alias "IpRenewAddress" ( _ AdapterInfo As IP_ADAPTER_INDEX_MAP) As Long
Private Declare Function GetNumberOfInterfaces Lib "IPHLPAPI.dll" ( _ ByRef pdwNumIf As Long) As Long
Private Declare Function GlobalAlloc Lib "kernel32" ( _ ByVal wFlags As Long, _ ByVal dwBytes As Long) As Long
Private Declare Function GlobalFree Lib "kernel32" ( _ ByVal hMem As Long) As Long
Private Declare Sub CopyMemory Lib "kernel32" Alias "RtlMoveMemory" ( _ Destination As Any, _ Source As Any, _ ByVal Length As Long)
Private Function IPOperation(ByVal dwAdapterIndex As Long, Optional OpCode As Integer = 0) As Boolean
Dim bufptr As Long Dim dwOutBufLen As Long Dim ip_map As IP_ADAPTER_INDEX_MAP
Dim ret As Long Dim nStructSize As Long Dim NumAdapters As Long Dim cnt As Long
If OpCode > 1 Or OpCode < 0 Then MsgBox "Invalid Operation code ", vbCritical: Exit Function End If
ret = GetInterfaceInfo(0, dwOutBufLen)
If ret <> 0 And ret = ERROR_INSUFFICIENT_BUFFER Then bufptr = GlobalAlloc(GMEM_FIXED, dwOutBufLen) ret = GetInterfaceInfo(bufptr, dwOutBufLen)
If ret <> ERROR_SUCCESS Then MsgBox "Error in retriving interface info": Exit Function End If
CopyMemory NumAdapters, ByVal bufptr, 4
nStructSize = LenB(ip_map)
If NumAdapters < 0 Then MsgBox "No Adapters found", vbExclamation: Exit Function End If
For cnt = 0 To NumAdapters - 1 CopyMemory ip_map, ByVal bufptr + (nStructSize * cnt) + 4, nStructSize
If ip_map.Index = dwAdapterIndex Then If OpCode = 0 Then ret = IPRenewAddress(ip_map) ElseIf OpCode = 1 Then ret = IPReleaseAddress(ip_map) End If If ret <> ERROR_SUCCESS Then MsgBox "Renew/Release Address error " & ret & ", Error# :" & Err.LastDllError End If
IPOperation = (ret = ERROR_SUCCESS) End If Next
End If
GlobalFree bufptr End Function
Sub ShowIPConfig() Dim AdapterInfoSize As Long Dim i As Integer, ret As Long Dim AdapterInfo As IP_ADAPTER_INFO Dim AddrStr As IP_ADDR_STRING Dim Buffer As IP_ADDR_STRING Dim pAddrStr As Long Dim pAdapt As Long Dim Buffer2 As IP_ADAPTER_INFO Dim AdapterInfoBuffer() As Byte
List1.Clear
AdapterInfoSize = 0 ret = GetAdaptersInfo(ByVal 0&, AdapterInfoSize) If ret <> 0 Then If ret <> ERROR_BUFFER_OVERFLOW Then MsgBox "GetAdaptersInfo sizing failed with error " & ret Exit Sub End If End If
ReDim AdapterInfoBuffer(AdapterInfoSize - 1)
ret = GetAdaptersInfo(AdapterInfoBuffer(0), AdapterInfoSize) If ret <> 0 Then MsgBox "GetAdaptersInfo failed with error " & ret Exit Sub End If
CopyMemory AdapterInfo, AdapterInfoBuffer(0), AdapterInfoSize pAdapt = AdapterInfo.Next
Dim cnt As Integer Do CopyMemory Buffer2, AdapterInfo, AdapterInfoSize List1.AddItem "[" & Buffer2.Index & "] " & TrimNull(Buffer2.Description)
List1.AddItem Space(5) & "IP : " & Buffer2.IpAddressList.IpAddress List1.ItemData(List1.NewIndex) = Buffer2.Index
pAddrStr = Buffer2.IpAddressList.Next
Do While pAddrStr <> 0 CopyMemory Buffer, Buffer2.IpAddressList, LenB(Buffer) List1.AddItem Space(5) & "IP : " & Buffer.IpAddress List1.ItemData(List1.NewIndex) = Buffer2.Index
pAddrStr = Buffer.Next If pAddrStr <> 0 Then CopyMemory Buffer2.IpAddressList, ByVal pAddrStr, LenB(Buffer2.IpAddressList) End If Loop
pAdapt = Buffer2.Next If pAdapt <> 0 Then CopyMemory AdapterInfo, ByVal pAdapt, AdapterInfoSize End If Loop Until pAdapt = 0 End Sub
Function FixStr(sMsg As String, Optional MaxLen As Integer = 25) As String Dim sPad As String Dim c As Long c = MaxLen - Len(sMsg) sPad = IIf(c > 0, Space(c), "")
FixStr = Left(sMsg, MaxLen) & sPad End Function
Private Function TrimNull(item) As String Dim pos As Integer pos = InStr(CStr(item), Chr$(0)) If pos Then TrimNull = Left$(item, pos - 1) Else: TrimNull = item End If End Function
Private Sub Command1_Click() Dim ret As Boolean If List1.ListIndex < 1 Then MsgBox "Please select the IP": Exit Sub If InStr(1, List1.List(List1.ListIndex), "IP") <= 0 Then MsgBox "Please select the IP": Exit Sub End If
ret = IPOperation(List1.ItemData(List1.ListIndex), 0) If ret = True Then MsgBox "Operation completed" ShowIPConfig End If
End Sub Private Sub Command2_Click() Dim ret As Boolean If List1.ListIndex < 1 Then MsgBox "Please select the IP": Exit Sub If InStr(1, List1.List(List1.ListIndex), "IP") <= 0 Then MsgBox "Please select the IP": Exit Sub End If
ret = IPOperation(List1.ItemData(List1.ListIndex), 1) If ret = True Then MsgBox "Operation completed" ShowIPConfig End If End Sub
Private Sub Form_Load() Dim cntInterfaces As Long Command1.Caption = "Renew" Command2.Caption = "Release" Call GetNumberOfInterfaces(cntInterfaces) If cntInterfaces > 1 Then ShowIPConfig Else Command1.Enabled = False Command2.Enabled = False End If End Sub |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
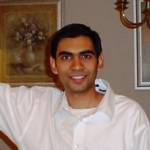
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|