|
|
|
The Win32 API allows binary files to be opened and written using the CreateFile, ReadFile, and WriteFile APIs. These functions offer increased flexibility to write and read from files. This article demonstrates a technique to write large amounts of data, in the form of a large array, to a binary file all at once instead of element by element.
Step-By-Step Example
- Create a standard exe project - Add the following code in form1 |
Click here to copy the following block | Const GENERIC_WRITE = &H40000000 Const GENERIC_READ = &H80000000 Const FILE_ATTRIBUTE_NORMAL = &H80 Const CREATE_ALWAYS = 2 Const OPEN_ALWAYS = 4 Const INVALID_HANDLE_VALUE = -1 Const FILE_NAME = "TEST.DAT"
Private Type MyType value As Integer End Type
Private Declare Function ReadFile Lib "kernel32" (ByVal hFile As Long, _ lpBuffer As Any, ByVal nNumberOfBytesToRead As Long, _ lpNumberOfBytesRead As Long, ByVal lpOverlapped As Long) As Long
Private Declare Function CloseHandle Lib "kernel32" ( _ ByVal hObject As Long) As Long
Private Declare Function WriteFile Lib "kernel32" ( _ ByVal hFile As Long, lpBuffer As Any, _ ByVal nNumberOfBytesToWrite As Long, _ lpNumberOfBytesWritten As Long, ByVal lpOverlapped As Long) As Long
Private Declare Function CreateFile Lib "kernel32" _ Alias "CreateFileA" (ByVal lpFileName As String, _ ByVal dwDesiredAccess As Long, ByVal dwShareMode As Long, _ ByVal lpSecurityAttributes As Long, _ ByVal dwCreationDisposition As Long, _ ByVal dwFlagsAndAttributes As Long, ByVal hTemplateFile As Long) _ As Long
Private Declare Function FlushFileBuffers Lib "kernel32" ( _ ByVal hFile As Long) As Long
Private Sub fillArray(anArray() As MyType) Dim x As Integer
For x = 0 To UBound(anArray) anArray(x).value = x Next x End Sub
Private Sub readArray(Fname As String, anArray() As MyType) Dim fHandle As Long Dim fSuccess As Long Dim sTest As String Dim lBytesRead As Long Dim BytesToRead As Long
BytesToRead = (UBound(anArray) + 1) * LenB(anArray(0)) fHandle = CreateFile(Fname, GENERIC_WRITE Or GENERIC_READ, _ 0, 0, OPEN_ALWAYS, FILE_ATTRIBUTE_NORMAL, 0) If fHandle <> INVALID_HANDLE_VALUE Then fSuccess = ReadFile(fHandle, anArray(LBound(anArray)), _ BytesToRead, lBytesRead, 0) fSuccess = CloseHandle(fHandle) End If End Sub
Private Sub writearray(Fname As String, anArray() As MyType) Dim fHandle As Long Dim fSuccess As Long Dim sTest As String Dim lBytesWritten As Long Dim BytesToWrite As Long BytesToWrite = (UBound(anArray) + 1) * LenB(anArray(0)) fHandle = CreateFile(Fname, GENERIC_WRITE Or GENERIC_READ, _ 0, 0, OPEN_ALWAYS, FILE_ATTRIBUTE_NORMAL, 0) If fHandle <> INVALID_HANDLE_VALUE Then fSuccess = WriteFile(fHandle, anArray(LBound(anArray)), _ BytesToWrite, lBytesWritten, 0) If fSuccess <> 0 Then fSuccess = FlushFileBuffers(fHandle) fSuccess = CloseHandle(fHandle) End If End If End Sub
Private Sub Form_Load() Dim fHandle As Integer Dim T(1000) As MyType Dim S(1000) As MyType
fillArray T writearray FILE_NAME, T MsgBox "Written to the disk" readArray FILE_NAME, S MsgBox "Read from the disk" End Sub |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
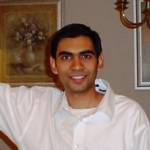
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|