|
|
|
Click here to copy the following block |
Option Explicit
Public Enum FindConditionConstants fccEqual fccNotEqual fccLess fccLessEqual fccGreater fccGreaterEqual fccInStr End Enum
Const DEFAULT_INITIALSIZE = 100
Const DEFAULT_CHUNKSIZE = 100
Private Type ListType Value As Variant prevNdx As Long nextNdx As Long End Type
Dim List() As ListType
Dim m_Count As Long
Private FirstNdx As Long Private LastNdx As Long
Private FreeNdx As Long
Private m_ChunkSize As Long
Private m_CurrIndex As Long
Private m_EOL As Boolean
Property Get CurrIndex() As Long CurrIndex = m_CurrIndex End Property
Property Let CurrIndex(ByVal newValue As Long) m_CurrIndex = newValue End Property
Sub MoveFirst() m_CurrIndex = FirstNdx End Sub
Sub MoveLast() m_CurrIndex = LastNdx End Sub
Sub MovePrevious() m_CurrIndex = List(m_CurrIndex).prevNdx m_EOL = False End Sub
Sub MoveNext() m_CurrIndex = List(m_CurrIndex).nextNdx m_EOL = True End Sub
Sub Move(ByVal Index As Long) CheckIndex Index m_CurrIndex = Index End Sub
Property Get BOL() As Boolean BOL = (m_Count = 0) Or (m_CurrIndex = 0 And m_EOL = False) End Property
Property Get EOL() As Boolean EOL = (m_Count = 0) Or (m_CurrIndex = 0 And m_EOL = True) End Property
Property Get Item(Optional ByVal Index As Long) As Variant Attribute Item.VB_UserMemId = 0 If Index = 0 Then Index = m_CurrIndex CheckIndex Index If IsObject(List(Index).Value) Then Set Item = List(Index).Value Else Item = List(Index).Value End If End Property
Property Let Item(Optional ByVal Index As Long, newValue As Variant) If Index = 0 Then Index = m_CurrIndex CheckIndex Index List(Index).Value = newValue End Property
Property Set Item(Optional ByVal Index As Long, newValue As Object) If Index = 0 Then Index = m_CurrIndex CheckIndex Index Set List(Index).Value = newValue End Property
Property Get IsEmpty() As Boolean IsEmpty = (m_Count = 0) End Property
Property Get Count() As Long Count = m_Count End Property
Function Add(Value As Variant, Optional ByVal Before As Long, _ Optional ByVal After As Long) As Long Dim ndx As Long Dim nextFreeNdx As Long If FreeNdx = 0 Then ExpandList m_ChunkSize ndx = FreeNdx nextFreeNdx = List(ndx).nextNdx If Before < 0 Then Before = FirstNdx If Before > 0 Then CheckIndex Before List(ndx).nextNdx = Before List(ndx).prevNdx = List(Before).prevNdx List(Before).prevNdx = ndx If Before = FirstNdx Then FirstNdx = ndx Else List(List(ndx).prevNdx).nextNdx = ndx End If ElseIf After > 0 Then CheckIndex After List(ndx).prevNdx = After List(ndx).nextNdx = List(After).nextNdx List(After).nextNdx = ndx If After = LastNdx Then LastNdx = ndx Else List(List(ndx).nextNdx).prevNdx = ndx End If Else If LastNdx Then List(LastNdx).nextNdx = ndx List(ndx).prevNdx = LastNdx Else FirstNdx = ndx List(ndx).prevNdx = 0 End If LastNdx = ndx List(ndx).nextNdx = 0 End If If IsObject(Value) Then Set List(ndx).Value = Value Else List(ndx).Value = Value End If m_Count = m_Count + 1 FreeNdx = nextFreeNdx Add = ndx End Function
Sub Remove(Optional ByVal Index As Long) If Index = 0 Then Index = m_CurrIndex CheckIndex Index If Index = FirstNdx Then FirstNdx = List(Index).nextNdx List(FirstNdx).prevNdx = 0 ElseIf Index = LastNdx Then LastNdx = List(Index).prevNdx List(LastNdx).nextNdx = 0 Else List(List(Index).prevNdx).nextNdx = List(Index).nextNdx List(List(Index).nextNdx).prevNdx = List(Index).prevNdx End If
List(Index).Value = Empty m_Count = m_Count - 1 If Index = m_CurrIndex Then m_CurrIndex = List(Index).nextNdx If m_CurrIndex = 0 Then m_EOL = True End If List(Index).nextNdx = FreeNdx List(Index).prevNdx = -1 FreeNdx = Index End Sub
Sub RemoveAll() Class_Initialize End Sub
Function Find(Value As Variant, Optional Condition As FindConditionConstants = _ fccEqual, Optional ByVal StartIndex As Long, Optional ByVal Descending As _ Boolean) As Long Dim isObj As Boolean If StartIndex = 0 Then StartIndex = m_CurrIndex ElseIf StartIndex < 0 Then If Not Descending Then StartIndex = FirstNdx Else StartIndex = LastNdx End If Else CheckIndex StartIndex If Not Descending Then StartIndex = List(StartIndex).nextNdx Else StartIndex = List(StartIndex).prevNdx End If End If isObj = IsObject(Value) Do While StartIndex If isObj Then If IsObject(List(StartIndex).Value) Then If Value Is List(StartIndex).Value Then If Condition <> fccNotEqual Then Exit Do Else If Condition = fccNotEqual Then Exit Do End If End If Else If Not IsObject(List(StartIndex).Value) Then Select Case Condition Case fccNotEqual If List(StartIndex).Value <> Value Then Exit Do Case fccLess If List(StartIndex).Value < Value Then Exit Do Case fccLessEqual If List(StartIndex).Value <= Value Then Exit Do Case fccGreater If List(StartIndex).Value > Value Then Exit Do Case fccGreaterEqual If List(StartIndex).Value >= Value Then Exit Do Case fccInStr If InStr(List(StartIndex).Value, Value) Then Exit Do Case Else If List(StartIndex).Value = Value Then Exit Do End Select End If End If If Not Descending Then StartIndex = List(StartIndex).nextNdx Else StartIndex = List(StartIndex).prevNdx End If Loop m_CurrIndex = StartIndex Find = StartIndex End Function
Sub SetSize(ByVal numEls As Long, Optional ByVal ChunkSize As Long) If numEls <= 0 Or ChunkSize < 0 Then Err.Raise 5 If numEls > UBound(List) Then ExpandList numEls - UBound(List) End If m_ChunkSize = ChunkSize End Sub
Private Sub Class_Initialize() ReDim List(0) As ListType ExpandList DEFAULT_INITIALSIZE m_ChunkSize = DEFAULT_CHUNKSIZE End Sub
Private Sub ExpandList(ByVal numEls As Long) Dim ndx As Long, newFreeNdx As Long newFreeNdx = UBound(List) + 1 ReDim Preserve List(UBound(List) + numEls) As ListType For ndx = newFreeNdx To UBound(List) List(ndx).nextNdx = ndx + 1 List(ndx).prevNdx = -1 Next List(UBound(List)).nextNdx = FreeNdx FreeNdx = newFreeNdx End Sub
Sub CheckIndex(ByVal Index As Long) If Index <= 0 Or Index > UBound(List) Then Err.Raise 5 If List(Index).prevNdx < 0 Then Err.Raise 5 End Sub |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
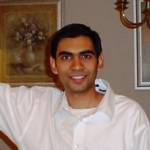
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|