|
|
|
If you are a VB6 programmer and want to learn VB.net then it will be easier for you but still you need to learn new language and syntax changes in VB.net. This article is for VB6 programmers who are moving to VB.net. New programmers can skip this article, we will cover each language feature in detail in "Language Features" article.
Option Statements
VB had option statements to allow us to override the default behavior of compiler. VB.net also supports the same concept. Following options are supported in VB.net
Option Explicit [On | Off] : If Value is [On] then you must declare variable before you use it. By enabling this option can prevent some common programming mistakes. Default value is [On].
Option Strict [On | Off] : If Value is [On] then automatic type casting won't happen means Integer variable can not be assigned to Long variable without using CLng(). Default value is [Off] so compiler will do automatic type casting for you.
Option Compare [Binary | Text] : If Value is [Binary] then strings are compared using binary compare algorithm. Default value is [Binary].
Option Base : This Option is no longer supported in VB.net
Note : You can apply module level option by adding Option statement on the very top. To enable Project Level Setting goto Project -> Properties -> Build.
Data Type Changes
In VB.net introduces several new datatypes which can affect VB6 programmers. Some of very important changes in datatypes are all native datatypes are now Object so now not only "real" Object is Object but native datatype like Integer, Boolean, String ... also Object. Another major change is datatype size change in Integer and Long datatype. Lets take a look in detail.
Integer type (Integer, Long, Short, Byte) changes - Byte (8 Bit) : This is unchanged in VB.net and can hold 0 to 255. - Short (16 Bit): This is new in VB.net. Short is same size datatype as VB6 Integer datatype. Short can hold -32,768 to +32,767 - Integer (32 Bit): Integer data type is changed in VB.net. In VB6 it used to be 2 Bytes but now its 4 Bytes. In VB.net Integer can hold -2,147,483,648 to +2,147,483,647 - Long (64 Bit): Long data type is changed in VB.net. In VB6 it used to be 4 Bytes but now its 8 Bytes. In VB.net Long can hold -9,223,372,036,854,775,808 to +9,223,372,036,854,775,807
Personally I liked Integer datatype change from 16 bit to 32 bit. Now VB.net Integer is consistent with SQL Server Int datatype and which will reduce many programming bugs.
Floating Point Division
VB.net still preserve the old Single and Double datatypes but divide by zero behaviour is different. Now the following code will note generate any error but it will show Infinity. |
This is bit surprising but it meets the IEEE standard.
Replacing Currency with Decimal
In VB6 Currency datatype was used to represent large floating point number but now no more Currency datatype in VB.net but you can use Decimal datatype which is 128 bit datatype.
Char Datatype
In VB6 we used to have Byte datatype to store 1 byte information. You can store anything between 0-255. You can also store single ASCII character but now we have another datatype "Char" which is 2 Bytes datatype so now you can not only store ASCII Character (1 Byte) but also you can store Unicode character (2 Byte).
Changes in String Datatype
VB.net has the same String datatype but internals are changed. In VB.net any alteration to String causes New string to be created. So the following statement will create new string instead of changing the existing string. |
This is surely performance hit but still its transparent to the developers.
Another change from VB6 to VB.net is now no more fixed length string. So the following statement is invalid in VB.net |
Variant datatype is gone
VB.net doesn't support Variant datatype but same thing is provided by Object datatype since every datatype is Object in VB.net.
CType statement
CType statement is a new casting feature in VB.net. CType can be used to cast object with one datatype to another datatype. CType also gives you design time intellisense. |
Declaration Changes
Multiple declaration
In VB.net you can declare multiple variables by specifying datatype once. In VB6 if you declare variable using Dim i,j,k,l as Integer then i,j,k are declared as Variant and only l is declared as Integer but now things has been changed in VB.net here is the example |
Declaring initial value
In VB.net you can set initial value while declaring the variable |
Dim as New
When you declare object using the following code in VB6 some weird things happen which you might not be aware of it. |
Here, it looks like object is created and memory is allocated because we used "New" keyword but that is not true. In VB6 object is not created until its accessed first time somewhere in the code....(Huhhhhh I didn't know that) Yes... VB6 compiler inserts object initialization code just before first time access of the object. This is surely performance hit. But now in VB.net you can expect what you see in your code ... means when you use "New" keyword object is initialized immediately.
Scoping Change
VB.net now supports block level scope for variable. Let's take a look at the following example |
The above code is invalid in VB6 but its totally valid in VB.net. VB6 only support Variable Scope upto Procedure level and VB.net supports block level scope means if you declare variable in a block (If...Else, Try...Catch, For...Next, While...Loop) your variable is valid only for that block.
Changes to Arrays
Zero based arrays
Array in VB.net is now zero based this means you can not declare array starting with 1 index which used to be in VB6. |
Declaring Array
Now in VB.net you can assign initial values when you declare your array |
Click here to copy the following block | Dim a1() As Integer = {1, 2, 3, 4, 5} Dim a2(,) As Object = {{100, "Honda"}, {101, "BMW"}, {102, "Toyota"}} |
Changes to User Defined Types
The concept of UDT (User defined type) allows you to create your own complex datatype. In VB6 you can write code as below to create Student datatype |
The same thing you can do in VB.net using "Structure" instead of "Type". VB.net doesn't support "Type" Keyword. Structure is more powerful and flexible then VB6 type. Structure can have their own sub/functions and properties just like a user defined Class but there is a clear difference between class and structure which we will see in detail in the "Language Features" chapter. |
Click here to copy the following block |
Public Structure SudentInfo Private m_ID As Integer Public Age As Integer Public FirstName As String Public Lastname As String Public Property ID() As Integer Get Return m_ID End Get Set(ByVal Value As Integer) If Value <= 0 Then m_ID = 0 Else m_ID = Value End If End Set End Property Public Function FullName() As String Return FirstName + " " + Lastname End Function End Structure |
Changes to Collections
VB6 had a collection datatype which was native. VB.net also supports the same functionality but now we have more classes to implement functionality like collection. All collection classes are in System.Collections namespace in VB.net and VB6 style collection is in Microsoft.VisualBasic namespace.
Here is the list of new collection classes in VB.net
- ArrayList : Implements single dimension array whose size grows dynamically as elements are added.
- BitArray : Implements a single dimension array of Boolean values which are stored internally as single bits, Providing a very compact way to organize list of Boolean values.
- HashTable : Implements a collection of Key-Value pairs that are organized based on hash value of the Key. This allows very fast and efficient storage and retrieval based on key value.
- Queue : Implements FIFO (First In First Out) structure
- Stack : Implements LIFO (Last In First Out) structure.
- SortedList : Implements a sorted list of Key-Value pairs
Now lets see the collection examples VB6 style collections and in VB.net style collections |
Click here to copy the following block | Sub FillVB6Col() Dim i As Integer Dim vb6Col As New Microsoft.VisualBasic.Collection For i = 0 To 1000000 vb6Col.Add("Item" & i, "Key" & i) Next End Sub
Sub FillVBNetCol() Dim i As Integer Dim vbnetCol As New System.Collections.Hashtable For i = 0 To 1000000 vbnetCol.Add("Item" & i, "Key" & i) Next End Sub |
Look at the performance difference between VB6 Collections and VB.net hashTable which gives you the same functionality as VB6 collection but the difference is huge in performance. Hashtable is quite faster than Microsoft.VisualBasic.Collection so try to avoid that in your code unless you have specific reason to use that.
New Arithmetic Operators
VB.net supports some new handy way to do common arithmetic operations. Here is the list of new arithmetic operators
Addition : i=i+5 ==> i+=5 Subtraction : i=i-5 ==> i-=5 Multiplication : i=i*5 ==> Division : i=i/5 ==> i/=5 Integer division : i=i\5 ==> i\=5 Exponent : i=i^5 ==> i^=5 String Concatenation : i=i & "Hello" ==> i&="Hello"
Bit Shift Operators
This is probabbly the most important operator change in VB.net.It is possible to write programs for your entire life and never need to use a bit shift operator, but if you are involved in mathematical programming, such as cryptography or graphics, then you will probably use them quite often. In addition to complex math, bit shifting is often used when working with older code, including the Win32 API, as developers would take a single larger data type, such as a 32-bit Integer value, and use that space to store multiple values, such as two 16 bit values.
Shifting to the Left or the Right There are two operators:
- << for shifting a specified number of bits to the left (towards the "high order" bits)
- >> for shifting to the right.
If a shift operation causes some number of bits to go outside of an underlying data type, then those bits are discarded. Empty bit positions created by the shift operation are always filled with 0s in a left shift operation and in a positive right shift operation. If a negative number of bit places is requested in a right shift operation (myNumber >> -4), then the vacated bit positions are filled with 1s.
The following diagram illustrates what happens when a 16-bit integer is shifted four places to the right. The four "low order" bits are discarded and the four "high order" bit positions are filled with 0s.
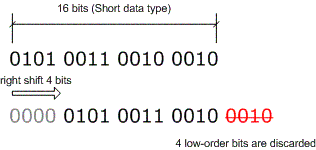
The above figure corresponds to the following Visual Basic .NET code: |
Breaking Apart a Multipart Value
The most common example to use shift operator is splitting R,G,B component from 32 bit value. Lets take a look at the folloing figure.
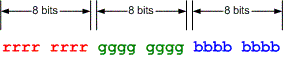
Now using shift operator you can write the following code to extract R, G and B |
Well above example was just to show how to use shift operator, actually we coule have done the exact same thing using inbulit .Net class System.Drawing.ColorTranslator which gives several methods to do color conversion.
No Set Statement
One of the most confusing syntax in the previous version of VB was Set statement. You use Set statement when assigning objects to variables. But now in VB.net no more confusion when you assign object to variables. Assignment is same as regular variable assignment. |
Changes to Property Routines
Since there is no Set statement in VB.net we can thing like there must be some changes with Property procedures too. In VB.net Property procedure now only contains Get and Set Procedures. No Let procedure in VB.net. |
Click here to copy the following block | Public Property Get Age() As Integer Age = m_Age End Property Public Property Let Age(Value As Integer) m_Age = Value End Property
Public Property Age() As Integer Get Age = m_Age End Get Set(ByVal Value As Integer) m_Age = Value End Set End Property |
You can see that in VB.net Property procedures are made consistent. VB.net also changes the ReadOnly, WriteOnly and Default property implementation. In VB6 to implement we only had to write Get procedure and to implement WriteOnly we had to implement Let or Set property but now things are more clear in VB.net. |
Click here to copy the following block | Public ReadOnly Property SSN() As String Get SSN = "666-66-6666" End Get End Property
Public WriteOnly Property Age() As Integer Set(ByVal Value As Integer) m_Age = Value End Set End Property |
Another change in Property routines is Default property. In VB6 you had to choose property type (Default) and there was no visible effect in the code so it was very obscure. Now things are much clear since you use Default keyword. |
Click here to copy the following block | Default Property Phone(ByVal PhoneType As String) As String Get Phone = colPhones.Item(PhoneType) End Get Set(ByVal Value As String) If colPhones.ContainsKey(PhoneType) Then colPhones.Item(PhoneType) = Value Else colPhones.Add(PhoneType, Value) End If End Set End Property
Dim myPerson As New Person myPerson("Home")="777-7777"
myPerson.Phone("Home")="777-7777" |
Structured Error Handling
This is one of the major changes in VB.net from VB6 world. VB6 was always criticized because of lacking feature for structured error handling. VB.net now supports Try..Catch..Finally Blocks to trap your error with in a specified block. Let's take a look at an example. |
Click here to copy the following block | Dim IsFileCopied As Boolean Try System.IO.File.Copy(strSourcePath, strDestPath) IsFileCopied = True Catch ex As Exception IsFileCopied = False Finally MsgBox("Is File Copied ? :" & IsFileCopied.ToString) End Try |
You can put any code between try and catch. If error occurs then any code in Catch block is executed. Code inside Finally block is always executed. You can also write multiple Catch block to trap different exceptions and take different actions on different errors. |
Click here to copy the following block | Dim IsFileCopied As Boolean
Try System.IO.File.Copy(strSourcePath, strDestPath) IsFileCopied = True Catch ex As System.IO.FileNotFoundException MsgBox("File not found") IsFileCopied = False Catch ex As Exception MsgBox("Unknown error") IsFileCopied = False Finally MsgBox("Is File Copied :" & IsFileCopied.ToString) End Try |
Even though we have new structured error handling in VB.net we can still use old "On Error GoTo errHandler" type code. You can also use "On Error Resume Next" but I would highly recommend to use Try..Catch error handling code. Since we have Try..Catch Block we have now "Exit Try" statement to quit code execution from the try catch block.
Procedure Changes
There are number of changes in VB.net how procedures (sub, functions and other methods) are called and created.
Parentheses are required on Procedure Calls
VB6 allowed you to call sub or function by just name but now its no more supported in VB.net. Parentheses are must while calling Sub or Function in Vb.net and also worth noting that while creating Object you need Parentheses.
ByVal Default for all Parameters
In VB6 most parameters were passed ByRef. Even though you don’t specify ByRef VB6 will pass all parameters ByRef means called procedure get actual reference of the parameter so any changes made to the parameters reflects back to the actual variable.
Now in VB.net by defauly all Parameters are pass ByVal so procedure gets a copy of parameters.
Optional Parameters Require Default Value
In VB6 we had optional parameter which is also true in VB.net but few things have been changed in VB.net. VB6 allowed you to define Optional Parameters of Variant type and you can use IsMissing function to check whether value is passed or not but in VB.net since there is no Variant datatype so we don't have IsMissing function. In VB.net you can not leave Optional Parameter without any default value.
Return Statement
In VB6 you used to return the value from function by simply assigning value back to function. i know this is bit confusing for who thing about recursion but that was the way how values were returned from function but now we have more clear way to do that. You can now use "Return" Statement to return the value from function. "Return" also exit the Sub, Function or Property.
Changes to Event Handling
Declaring events and firing events from class is same as VB6 but handing events is different to VB6 developer. |
Click here to copy the following block | Private WithEvents myObj As MyClass Private Sub myObject_MyEvent(strMsg As String) MsgBox strMsg End Sub
Private WithEvents myObj As MyClass Private Sub MyEventHandler(ByVal strMsg As String) Handles myObj.MyEvent MsgBox (strMsg) End Sub |
So only difference in VB6 and Vb.net is "Handles" keyword and in Vb.net you can have any name as your Event Sub (Event Handler). VB.net also allows you to handle multiple events using one Event handler routine as shown below. |
Click here to copy the following block | Private WithEvents myObj1 As MyClass Private WithEvents myObj2 As MyClass Private Sub MyEventHandler(ByVal strMsg As String) Handles myObj1.MyEvent1, myObj2.MyEvent1, myObj2.MyEvent2 MsgBox (strMsg) End Sub |
Conclusion
So far we have gone through couple of important changes in VB.net for VB6 programmer’s point of view. I haven't covered all changes and new features in VB.net but you will learn as you walk through the tutorial series. I would like to hear from you about this article. |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
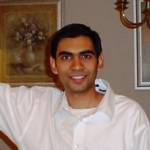
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|