|
|
|
Introduction
This tutorial series is intended to initiate the reader into the world of .NET. I will try to cover most of the important .Net features. This tutorial will be useful for anyone with a programming background, especially for those who know VB.
There are many great additions in .Net. I think VB programmers will be very happy to find all those great features which were seriously missing in VB. I am talking about features like Threading, Inheritance, Polymorphism, object oriented programming approach like C++ and list goes on and on. Also, there are many other features which they had to implement using workarounds till now. If you dont have lots of time to go through one of those huge books to learn .Net, then this tutorial series is for you. It is a quick learning guide with fully working sample programs and screenshots.
What Is the Microsoft .NET Framework?
To put in simple words, ".Net Framework" is a set of class libraries which developers can use to develop programs which can be executed under the full control of a managed environment known as CLR (conceptually similar to Java Virtual Machine). The .NET Framework consists of three main parts: the Common Language Runtime (CLR), a hierarchical set of unified class libraries, and a componentized version of Active Server Pages called ASP.NET.
Now let’s understand little more about .Net Framework. The .NET Framework encompasses the following:
A new way to expose operating system API's and other Class Libraries - What this means is that in .Net most of time you don’t have to worry about writing lots of code to accomplish various tasks which you used to do with APIs in VB6. The .Net Framework comes with hundreds of classes as a part of .Net Framework. Some good examples are Security Classes, Threading Classes, Graphics Classes, Networking Classes and many more all these are now part of .Net framework (Wooooow - Can't believe this - Too good!).
A new infrastructure for managing application execution -the Common Language Runtime) - The biggest problems in old programming approach were memory management and security which are gone with .Net Framework. The .Net Framework comes with inbuilt memory management mechanism known as garbage collection so you don’t have to worry to clean your memory when you exit the program .Net Framework will handle it for you. Moreover Microsoft developed a new runtime environment known as the Common Language Runtime (CLR). The CLR includes the Common Type System (CTS) which means all different languages C#, VB.net, J# use the same data types which gives you cross-language class Inheritance, cross-language type compatibility and many great features.
Microsoft Intermediate Language - To support hardware and operating-system independence, Microsoft developed the Microsoft Intermediate Language (MSIL, or just IL). IL is a CPU-independent machine language-style instruction set into which .NET Framework programs are compiled. IL programs are compiled to the actual machine language on the target platform prior to execution (known as just-in-time(JIT) compiling). IL is never interpreted. IL is a concept very similar to ByteCode in JAVA and CLR concept is very similar to JVM (Java Virtual Machine).
A new web server paradigm - The biggest change in Web Development is that now ASP.net is fully compiled instead of interpreted. Classic ASP is interpreted so much slower than ASP.net. Now ASP.net can use fully Object Oriented Programming Model.
A new focus on distributed-application architecture - In DotNet Microsoft has tried give more and more features to make robust Distributed Applications. XML Webservices, Remoting, ADO.net - all these new technologies clearly tell you where Microsoft wants to go using the powerful .Net Platform.
Million times asked Question: C# or VB.net?
I have been asked several times - which language is more powerful ? Or which language should I choose?... C# or Vb.net? I always answer - "It depends..."
All .Net languages use the same base libraries and CTS (Common Type System) therefore there is no major difference in functionality. So in the .NET family, VB.net is as powerful as C# ! If you are coming from a VB6 background then you might find yourself more convenient with VB.net and if you are C/C++ or JAVA programmer then you will feel more comfortable with C#. So, its probably only a matter of choice!
Why do we need Microsoft .Net?
I used to work with VB6, I really liked VB6 but it had its own limitations. Here I am not only talking about VB6 but overall software development for Windows. When we start software development the most important things are
- Development Time/Cost
- Software Maintenance Cost
- Application Scalability/Stability
- Testing/Debugging
- Setup/Deployment
VB6 belonged to the RAD (Rapid Application Development) paradigm, but RAD means compromises - VB6 lacks some serious enterprise application development features (i.e. Full Object Oriented Capabilities, Threading, easy Setup/Deployment …). Dotnet framework provides hundreds of inbuilt class libraries (e.g. Threading, IO, Networking, Security etc.) which can be used by windows programmers to write very efficient code. And all this without losing RAD capabilities! Some of the GOLD features in VB.net for VB6 Programmers are
- Structured Error handling
- Threading Support
- Full Inheritance and other OO features
- Powerful windows form designer (WinForm designer)
- Setup/Deployment without any 3rd party tool
You can get full power of .Net technology using world’s best IDE Visual Studio 2003 and now there is already a beta release of Visual Studio 2005 (Codename: Whidbey).
Your very first “Hello World” program in VB.net
Now lets implement our first "Hello World" VB.net Program. There are several changes in VB.net from VB6. Visual Studio.Net IDE has been totally redesigned but still many things are same like it was in VB6.
Open Visual Studio.net and Click on the "New Project" from the start page or File Menu->New->Project.
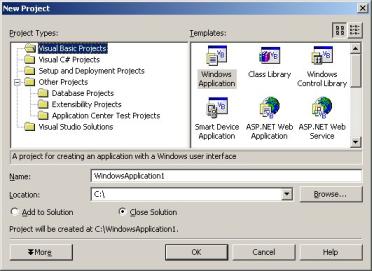
On the New Project Dialog select Windows Application. Now Visual Studio will open a defult form1 just like VB6 IDE does. But wait its not quite similar to VB6.... yes in DotNet you will see lots of code generated by IDE but you dont have to worry about that at all. Any time you place a control or change design time properties WinForm designer adds/changes code for you. Lets see more...
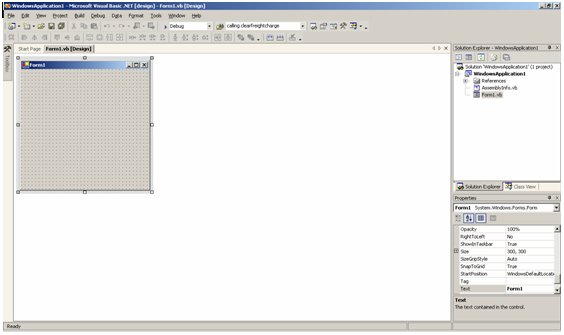
Now let's place a Command Button control on the form1. Expand toolbox from left hand side. Drag Command Button control from "Windows Form" tab from Toolbox. Double click on the CommandButton1 and it will show you the Code window as shown below.
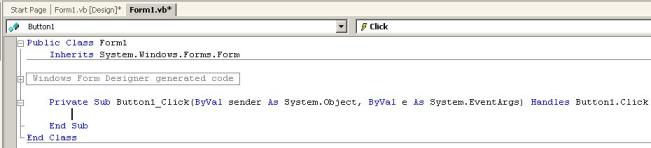
Enter the code shown as below in the Button1 Click event. |
Click here to copy the following block | Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click MsgBox(strMessage, MsgBoxStyle.Information, strTitle) End Sub |
Before we run the demo lets look at the code in IDE. Since we haven't declared variables (strMessage and strTitle) IDE will indicate by showing you lines under the non declared variables. Take your mouse over it and you will see error description as shown below.

Now Press F5 to run the project. Actually we are missing variable declarations, so it will throw errors but here werun it intentionally so that we can see some cool features of Visual Studio.net IDE. After you run the project it will fail to compile because we didn't declare two variables. Visual Studio IDE will show all errors in the task list as shown below. The good thing about VB.net is you will get notified for all errors at once when you compile it which is essential when you are dealing with a large project. Once you correct the error displayed in the "task list" it should automatically disappear from the list. You can also double-click on the error to locate it in the current project.
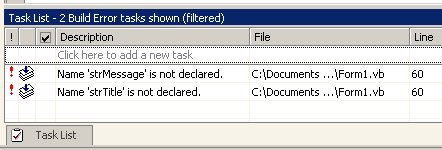
Ok now lets correct our error by declaring variables and assigning some values. |
Click here to copy the following block | Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click Dim strMessage As String = "Hello World" Dim strTitle As String = "Binaryworld" MsgBox(strMessage, MsgBoxStyle.Information, strTitle) End Sub |
Now press F5 to run the project or click Debug Menu->Start and then click on the button1 to see your very first "Hello World" in VB.net using Visual Studio.net
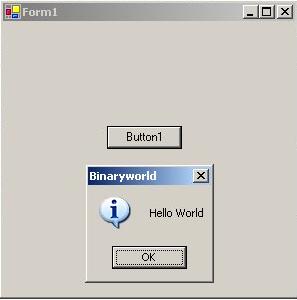
Summary
In this article I've given you the basic introduction to Microsoft .net technology and you also learned what the .Net Framework is and how you can take advantage of this exciting technology. We created the first "Hello World" program in VB.net and we also saw some kool IDE features. |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
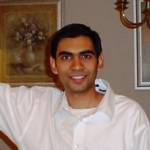
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|