|
|
|
.NET strings are objects, so what you store in a String variable is actually a reference to a String object allocated in the managed heap. Even though the VB.NET compiler attempts to hide the object nature of strings as much as it can - for example, you don't need to declare a String object with a New operator - in many cases being aware of their object nature can help you in making the most out of strings, as well as avoid some subtle programming mistakes. For example, consider this code: |
The above code is the kind of code that a VB6 developer would write, but it can be improved remarkably by recognizing that strings are objects. In fact, the Res variable contains either a reference to the same object pointed to by the s1 variable or the object pointed to by the s2 variable, so you can make the test faster by using the Is operator: |
Because the VB.NET compiler creates a single string object for all the string constants that have the same value, the same kind of optimization can be applied when constant strings are involved. Consider this code: |
Click here to copy the following block | Dim color As String
Select Case New Random().Next(0, 2) Case 0 : color = "Black" Case 1 : color = "White" Case Else : color = "Unknown" End Select
If color Is "Black" Then ElseIf color Is "White" Then Else End If |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
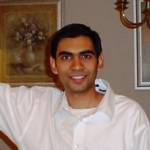
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|