|
|
|
In a typical user registration form, you may ask for quite a lot of data, such as the first and last name, full address, phone and fax numbers, e-mail address, newsletters subscriptions and other data for the site personalization. All this data shouldn't the asked in a single step, because a long list of input controls would probably discourage an user. It would be better to divide the form in a few steps, at least 2 or 3, so that the user perceives the process as simpler (even though they will ultimately fill in the same input controls anyway, of course). To do this, the typical ASP approach is to write separate pages, and pass the control from one to the following page. However, this is not very straighforward for the programmer, because he must find a way to pass to the following page all the user data typed into the current form, so that at the end of the process the last page will get all the inserted user data. Passing user data from a page to another is usually done with parameters in the querystring or with session variables, but in this case you can avoid these methods at all, and use a totally different approach to create multi-steps input forms. You can create two or three panels on the ASPX page, and show/hide them (by means of the Visible property) according to the current step index (that in increased/decreased by clicking two buttons). The Panel control acts like a container control, so you place the input controls of each steps inside the respective panel. Here's how you define the panels in the ASPx page: |
Click here to copy the following block | <form id="Form1" method="post" runat="server"> <asp:Panel Runat="server" ID="FirstStep"> <!-- put here the input controls for the first step --> </asp:Panel> <asp:Panel Runat="server" ID="SecondStep" Visible="False"> <!-- put here the input controls for the second step --> </asp:Panel> <asp:Panel Runat="server" ID="ThirdStep" Visible="False"> <!-- put here the input controls for the third step --> </asp:Panel> <br> <asp:Button Runat="server" ID="Previous" Visible="False" Text="Previous" _ Width="60px" /> <asp:Button Runat="server" ID="Next" Text="Next" Width="60px" /> <asp:Button Runat="server" ID="Finish" Text="Finish" Visible="False" Width= _ "60px" /> </form> |
Note that only the first panel is visible by default, the other two are hidden. When the user presses the Next button, the first panel and its child controls will be made invisible, and the second panel will be shown with its child controls. The process is similar for the third step, where also a Finish button will be made visible. The great thing of this approach is that you don't need to have a separate page for each step, and so don't need to pass values from a page to another. Also consider that when a control has its server-side Visible property set to False its content is not sent to the client browser at all, so if you use this method you won't be sending the HTML code for the whole page, but just the HTML for the current step. Here's how you manage the whole process from the code-behind class: |
Click here to copy the following block | Public Class InputForm : Inherits System.Web.UI.Page Protected FirstStep As System.Web.UI.WebControls.Panel Protected SecondStep As System.Web.UI.WebControls.Panel Protected ThirdStep As System.Web.UI.WebControls.Panel Protected ThirdStep As System.Web.UI.WebControls.Panel Protected Previous As System.Web.UI.WebControls.Button Protected Finish As System.Web.UI.WebControls.Button Protected Next As System.Web.UI.WebControls.Button
Private Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) If Not Me.IsPostBack Then ' the first time the page is loaded, set the current step index to 1 ViewState("CurrentPage") = 1 End If End Sub
Private Sub Previous_Click(ByVal sender As Object, _ ByVal e As System.EventArgs) ' Previous button clicked, decrease the step index by 1 ShowPage(Integer.Parse(ViewState("CurrentPage").ToString()) - 1) End Sub
Private Sub Next_Click(ByVal sender As Object, ByVal e As System.EventArgs) ' Next button clicked, increase the step index by 1 ShowPage(Integer.Parse(ViewState("CurrentPage").ToString()) + 1) End Sub
Private Sub ShowPage(ByVal page As Integer) ' save the current step index in the ViewState ViewState("CurrentPage") = page
' show/hide the panels and the Next/Previous/Finish buttons according ' to the current step index Select Case page Case 1 Previous.Visible = False Next.Visible = True FirstStep.Visible = True SecondStep.Visible = False Exit Sub Case 2 Previous.Visible = True Next.Visible = True Finish.Visible = False FirstStep.Visible = False SecondStep.Visible = True ThirdStep.Visible = False Exit Sub Case 3 Next.Visible = False Previous.Visible = True Finish.Visible = True SecondStep.Visible = False ThirdStep.Visible = True Exit Sub End Select End Sub
Private Sub Finish_Click(ByVal sender As Object, _ ByVal e As System.EventArgs) ' When the Finish button is clicked, process the user input End Sub End Class |
|
|
|
Submitted By :
Nayan Patel
(Member Since : 5/26/2004 12:23:06 PM)
|
 |
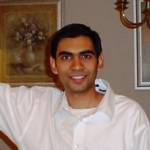
|
Job Description :
He is the moderator of this site and currently working as an independent consultant. He works with VB.net/ASP.net, SQL Server and other MS technologies. He is MCSD.net, MCDBA and MCSE. In his free time he likes to watch funny movies and doing oil painting. |
View all (893) submissions by this author
(Birth Date : 7/14/1981 ) |
|
|